Tips to build a Python Chatbot using a Chatbot API
Python and chatbot are going through a love story that might be just the beginning. Many companies choose to create chatbots using Python for many reasons and sometimes, just because of the hype.
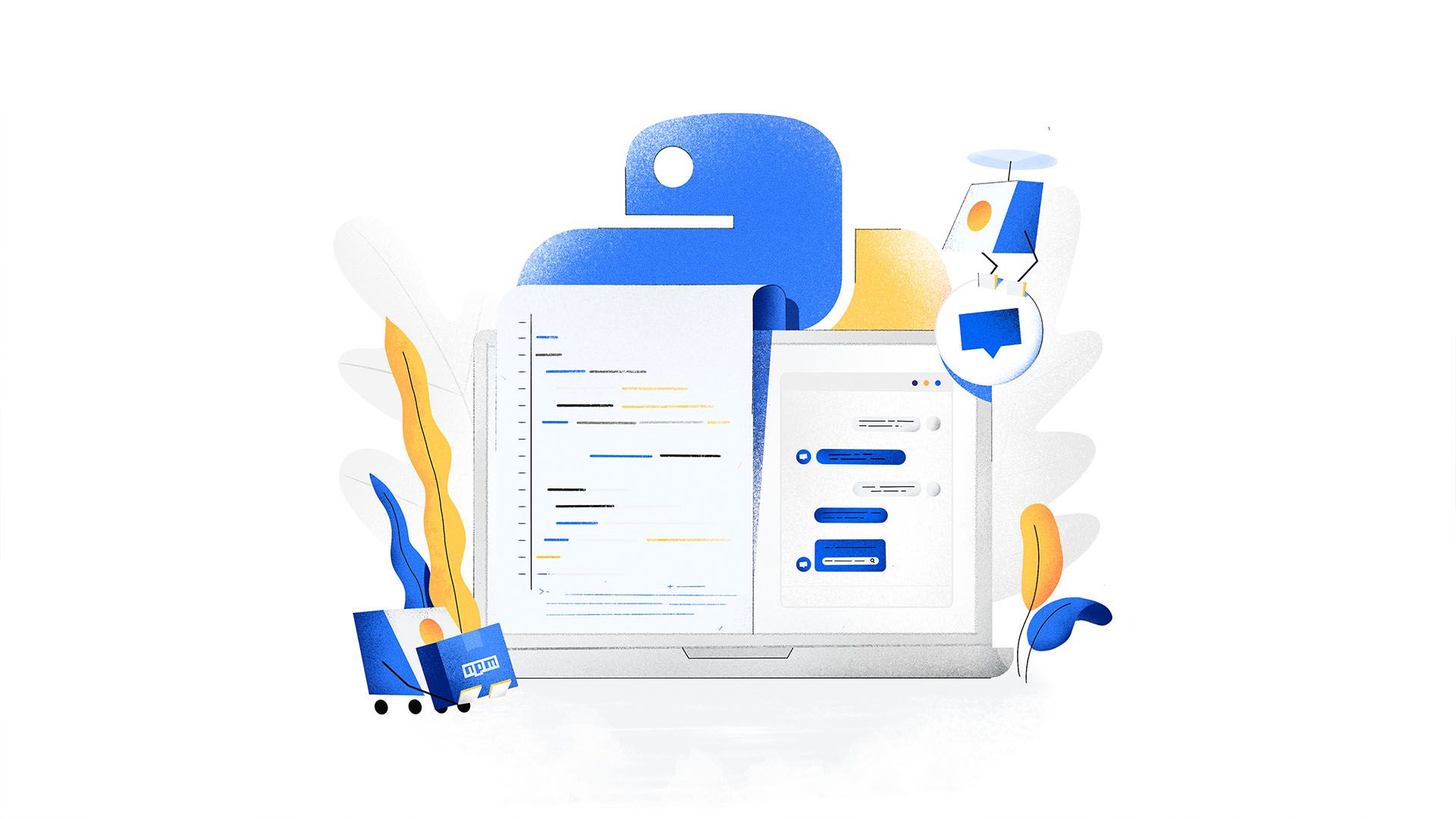
Python and chatbot are going through a love story that might just be the beginning. Many companies choose to create chatbots using Python for many reasons and sometimes, just because of the hype.
Through this quick article, we will give you our best tips to not miss the steps on your way to build the best conversational experience.
As multiple tools now offer the ability to build chatbots using Python, as we do at crisp with our chatbot API, it is easier than ever to create the greatest customer experience, in just a few lines of code.
No matter you build an AI chatbot or a scripted chatbot, Python can fit both.
Why is Python trending?
There is a lot of hype around Python at the moment, especially. when talking about conversational experiences.
1. Python Has a Healthy, Active, and Supportive Community
Python has been around for a while, so there’s plenty of documentation, guides, tutorials, and more. Plus, the developer community is incredibly powerful. That means any time someone has a question, they can get an answer in a little to no delay.
2. Python Has Some Great Corporate Sponsors
Google adopted Python back in 2006, and they’ve used it for many platforms and applications since.
Why does this matter? Because if companies like Google want their team — and future developers — to work with their systems and apps, they need to provide resources. In Google’s case, they created a vast quantity of guides and tutorials for working with Python.
For example, you can follow this free Python class that has been created by Google.
3. Python Offers Big Data Solutions
The use of big data and cloud computing solutions has also helped skyrocket Python to what we know. It is one of the most popular languages used in data science, second only to R. It’s also being used for machine learning and AI systems and various modern technologies.
Of course, it helps that Python is incredibly easy to analyze and organize into usable data.
4. Python Is Reliable and Efficient
Ask any Python developer — or anyone that has ever used the language — and they’ll agree it’s strong, reliable, and efficient. You can work with and deploy Python applications in nearly any environment, and there’s little to no performance loss no matter what platform you work with.
Again, because it’s versatile, this also means you can work across several domains including — but not limited to — web development, desktop applications, mobile applications, hardware, and more.
Building a chatbot is one of the main reasons you'd use Python. Here are a few tips not to miss when combining a chatbot with a Python API.
The boundaries of a chatbot
When you begin to work on a conversational experience, even a trivial one, you’ll need to answer those fundamental questions:
- Domain knowledge: What does a user expect this bot to understand?
- Personality: What tone or vocabulary does the bot employ?
Domain knowledge
True artificial intelligence does not exist, so while some AIs can imitate humans or answer some kinds of factual questions, all chatbots are restricted to a subset of topics. IBM's Jeopardy-playing Watson “knew” facts and could construct realistic responses, but it couldn’t schedule your meetings or deliver your last shopping sesh. Simple sales bots like SlackBot or CrispBot can successfully help users setup their accounts but aren’t designed to engage you in open-ended dialogue.
Personality
Bots have historically been personalized as something less than human to excuse their bad responses and frustrating lack of comprehension. This can be an opportunity for creativity and funny invention. It’s can be disappointing that so many bots are personified as females or teenagers, as if those groups were naturally not fully human. But when engaging a conversation, it's always better for a bot to try to behave like a human so the conversation has a better-perceived value.
Often the dual axes of domain and personality align but domain and personality don’t necessarily need to be tightly coupled, though—an ecommerce bot needs to know about products, sizing, and order status, but that domain doesn’t imply any particular kind of personality. A shopping bot could have the persona of a helpful person, a cheerful kitten, or have no personality at all.
That's why combining personality and domain knowledge can add a little bit of value in your customers' experience.
To build a great chatbot using Python, here is our Python API Wrapper.
What are the best libraries to build a chatbot using Python?
Before building your next bot, it's great to step back and think about the library you're going to use to create a natural conversation over the chat.
Here are some of the best NLP libraries we can advise:
Natural Language ToolKit
NLTK is a leading platform for building NLP programs to work with human language data. It provides easy-to-use interfaces to over 50 corpora and lexical resources such as WordNet, along with a suite of text-processing libraries for classification, tokenization, stemming, etc. This library provides a practical introduction to programming for language processing.
This is one of the best libraries available at the moment.
Gensim
Gensim is a Python library for topic modeling, document indexing, and similarity retrieval with large corpora. The target audience is basically the natural language processing (NLP) and information retrieval (IR) community.
This algorithm isn't specialized in NLP but can be pretty much efficient and a bit more exhaustive than NLTK.
Polyglot
Polyglot is a natural language pipeline that supports massive multilingual applications. The features include tokenization, language detection, named entity recognition, part of speech tagging, sentiment analysis, word embeddings, etc. Polyglot depends on Numpy and libicu-dev, on Ubuntu/Debian Linux distribution that you can use over those OS.
Those 3 libraries are really powerful but there are more interesting solutions that can be added to your chatbot when building an AI chatbot.
Dialogflow
Dialogflow isn't a Google library. It's a dedicated tool, aimed at doing the NLP job on his own.
It gives users ways to interact with your product by building engaging voice and text-based conversational interfaces, such as voice apps and chatbots, powered by AI. Connect with users on your website, mobile app, the Google Assistant, Amazon Alexa, Facebook Messenger, and other popular platforms and devices.
As you see, it goes way further than a simple chatbot.
Tensorflow
TensorFlow is an end-to-end open source platform for machine learning. It has a comprehensive, flexible ecosystem of tools, libraries and community resources that lets researchers push the state-of-the-art in ML and developers easily build and deploy ML powered applications.
It's also much more than a platform dedicated to chatbot but can be very powerful.
But tools are not everything, here are our best tips to take advantage of a Python API to build chatbots.
How to build a simple chatbot using Python in few minutes
We will create a simple chatbot using Wit.ai and Crisp to order a burger
Wit.ai will be used as a NLP processor in order to convert to convert user text queries into a computer readable queries.
Finally, we use Crisp for our chat interface.
1. Configure Wit.AI
First, we will create an account on Wit.AI and then create an intent called "Order burger"
Once we created this intent, we can train it with custom sentences like "I would like to order a burger"
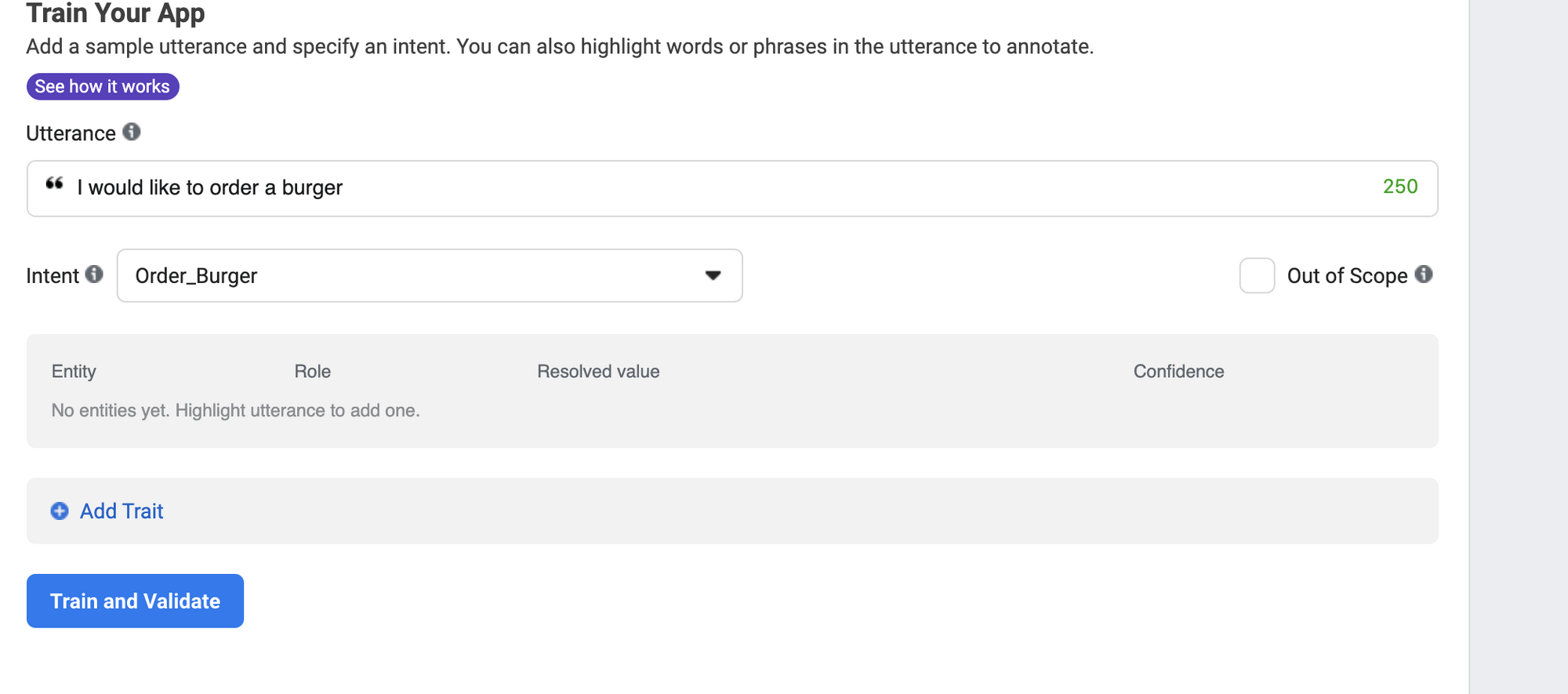
Once this intent is configured, Wit.ai will be able to understand other sentences like "I want to eat an hamburger"
Finally, we will need to get our server access token:
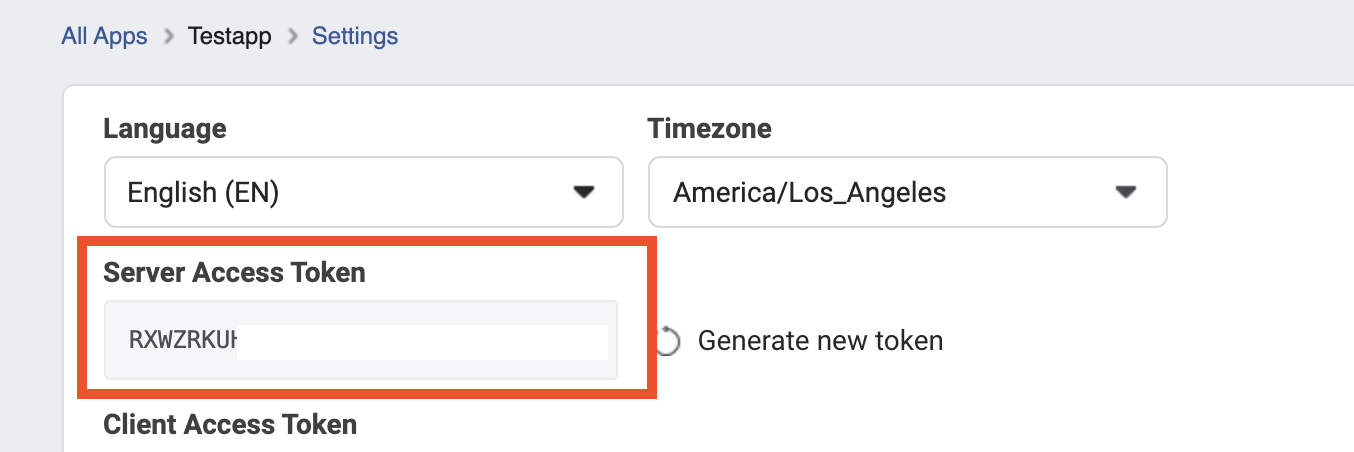
2. Configuring Crisp
We will need to create an account on Crisp. Once we created our account on Crisp, we will need to retrieve our live chat code. It is a javascript snippet you can embed on an HTML page.
To retrieve your website code:
- Go to https://app.crisp.chat/settings/websites/, then click integrations

- Go to HTML
- Copy the script tag
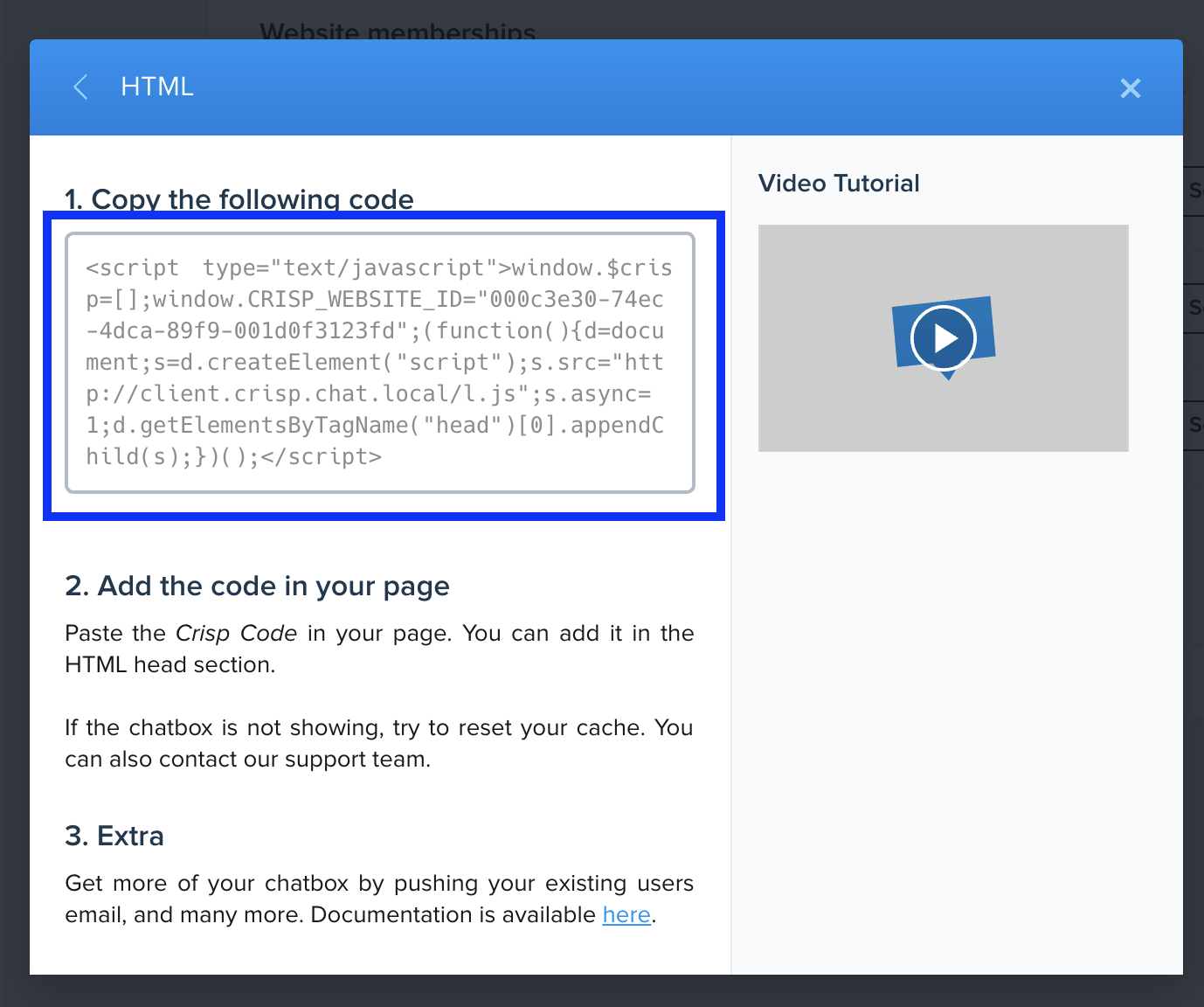
We can then embed the script tag in our HTML.
3. Building a basic chatbot using Python
We will create a very simple python server that listens to requests using a POST Request.
from http.server import HTTPServer, BaseHTTPRequestHandler
from io import BytesIO
class SimpleHTTPRequestHandler(BaseHTTPRequestHandler):
def do_GET(self):
self.send_response(200)
self.end_headers()
self.wfile.write(b'<script>//PASTE Your widget code here</script>')
def do_POST(self):
content_length = int(self.headers['Content-Length'])
body = self.rfile.read(content_length)
print(body)
self.send_response(200)
self.end_headers()
httpd = HTTPServer(('localhost', 8000), SimpleHTTPRequestHandler)
httpd.serve_forever()
We can then start this script using
python server.py
Finally, we can use Ngrok to make allow Crisp to send webhooks to our script
./ngrok http 8000
Then, we can configure Crisp to send webhooks to our custom script:
To do that we need to got to:
- https://app.crisp.chat/settings/websites/
- Click your website settings
- Advanced configuration
- Web hooks
- Click "Add a web hook"
- Add the URL generated by Ngrok
- Enable message:send
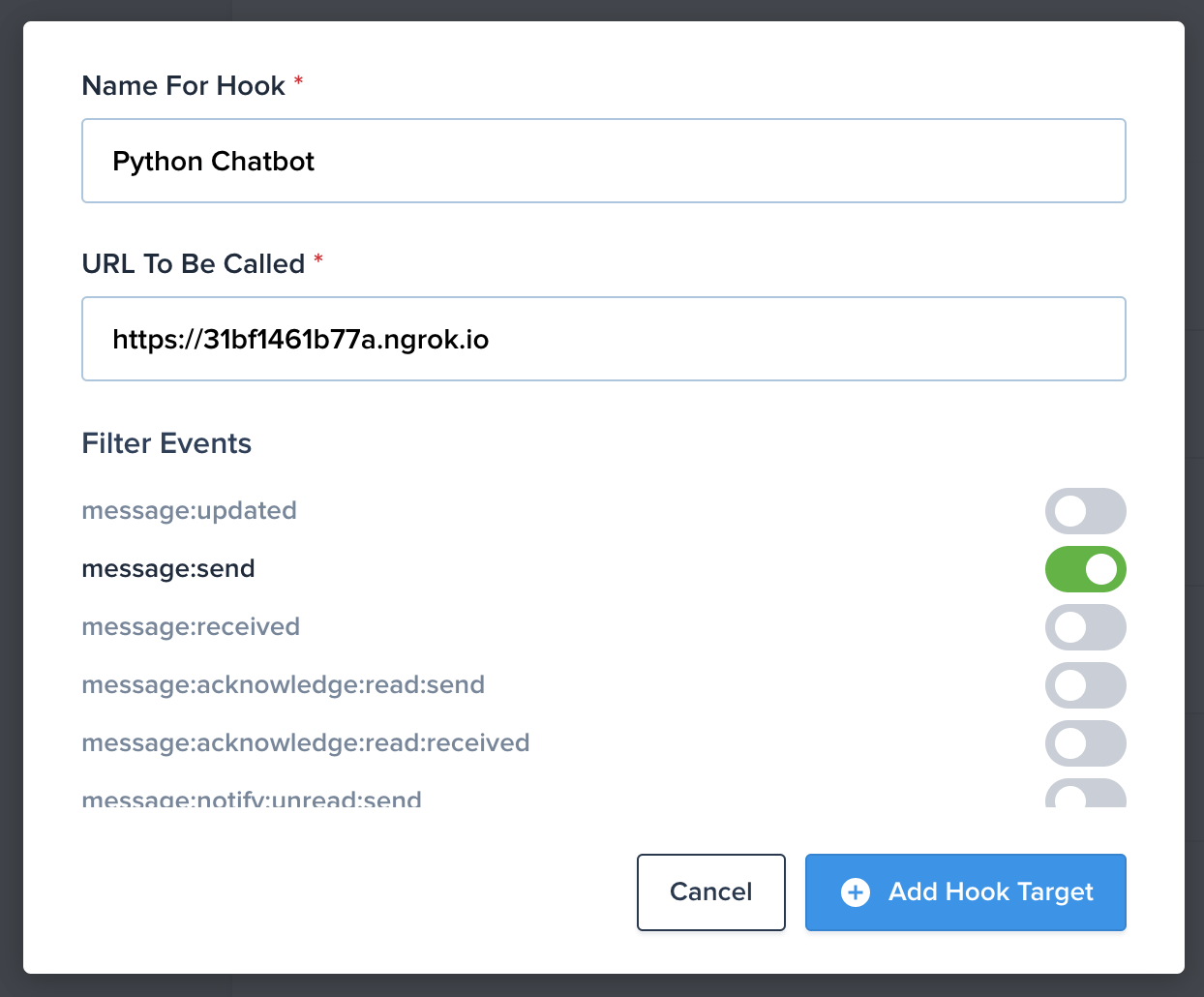
We can now go http://localhost:8000 and send our first message
As we can see, we just received a message from our chatbot! Now we need to craft a reply

4. Reply to user requests
In order to reply to user requests, we will need to install the crisp python API using https://github.com/crisp-im/python-crisp-api
pip install crisp-api
Then import it in our code
from crisp_api import Crisp
Then we can get our Crisp API credentials using this link: https://marketplace.crisp.chat/
This way we can your token identifier and token key:
client = Crisp()
client.authenticate(identifier, key)
Finally we also need to parse Crisp webhook using JSON. To do that we import the json library
import json
Now, we can reply to our user message request using the Crisp API
payload = json.loads(body)
client.website.send_message_in_conversation(payload["data"]["website_id"], payload["data"]["session_id"], {
"type": "text",
"content": "This message was sent from python-crisp-api! :)",
"from": "operator",
"origin": "chat"
})
Finally, we can send a dummy chat message from http://localhost:8000
And finally, we get a reply from our chatbot

5. Using Wit.ai to conditionally reply to user messages
We can now import Wit.Ai python API in our project
pip install wit
And finally we can parse the user message content using:
from wit import Wit
wit_client = Wit("YOUR_SERVER_TOKEN")
wit_ai_data = client.message(payload["data"]["content"])
print(wit_ai_data)
You will need to replace YOUR_SERVER_TOKEN with the server token from Wit.AI dashboard.
Finally, we can send a test message from http://localhost:8000
Wit.AI replies:
{'text': 'Order a burger', 'intents': [{'id': '333607671262134', 'name': 'Order_Burger', 'confidence': 1}], 'entities': {}, 'traits': {}}
It is now possible to reply using the chatbot only if the Intent is burger:
if wit_ai_data["intents"][0]["name"] == "Order_Burger":
client.website.send_message_in_conversation(payload[
"data"]["website_id"], payload[
"data"]["session_id"], {
"type": "text",
"content": "Would you like to order a burger?",
"from": "operator",
"origin": "chat"
})
6. Complete example
Here is what your code should look like:
from http.server import HTTPServer, BaseHTTPRequestHandler
from io import BytesIO
from crisp_api import Crisp
from wit import Wit
import json
class SimpleHTTPRequestHandler(BaseHTTPRequestHandler):
def do_GET(self):
self.send_response(200)
self.end_headers()
self.wfile.write(b'<script type="text/javascript">window.$crisp=[];window.CRISP_WEBSITE_ID="XXXXXXXXX";(function(){d=document;s=d.createElement("script");s.src="https://client.crisp.chat/l.js";s.async=1;d.getElementsByTagName("head")[0].appendChild(s);})();</script>')
def do_POST(self):
content_length = int(self.headers['Content-Length'])
body = self.rfile.read(content_length)
client = Crisp()
client.authenticate("XXXXXXXX", "XXXXXXXXX")
payload = json.loads(body)
wit_client = Wit("XXXXXXXX")
wit_ai_data = wit_client.message(payload["data"]["content"])
if wit_ai_data["intents"][0]["name"] == "Order_Burger":
client.website.send_message_in_conversation(payload[
"data"]["website_id"], payload[
"data"]["session_id"], {
"type": "text",
"content": "Would you like to order a burger?",
"from": "operator",
"origin": "chat"
})
self.send_response(200)
self.end_headers()
httpd = HTTPServer(('localhost', 8000), SimpleHTTPRequestHandler)
httpd.serve_forever()